The Sneaky Switch Statement: JavaScript Interview Tips (Part 2)
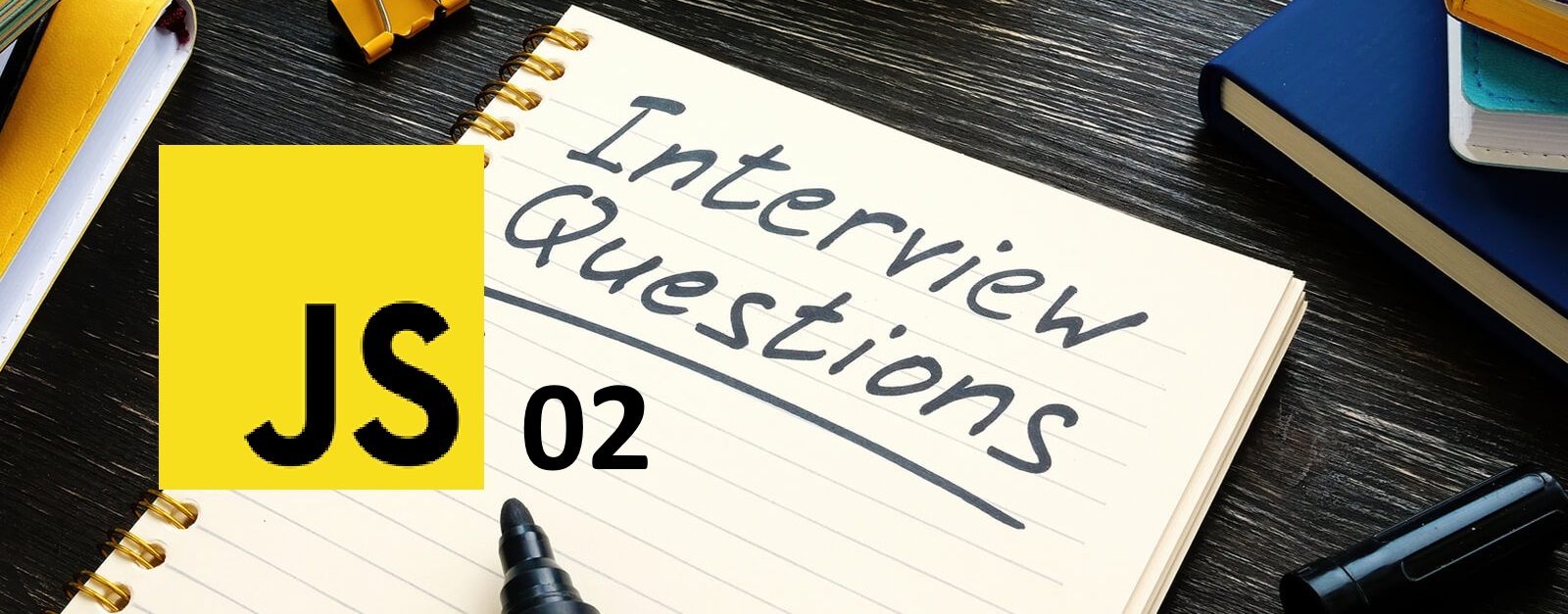
Hey there, code adventurers! π Ready to unravel a JavaScript mystery that’s been known to make even seasoned developers scratch their heads? Let’s jump into the world of switch statements, type coercion, and the quirks that make JavaScript… well, JavaScript!
The Code That Started It All
First, let’s revisit our tricky little code snippet:
javascript var B = 65; // ASCII value of A
var grade = B;
var result = 0;
switch(grade) {
case 'A': {
result += 10;
break;
}
case 'B': {
result += 9;
break;
}
case 'C': {
result += 8;
break;
}
default:
result += 0;
}
document.write(result);
Now, you might be thinking, “65 is ‘A’ in ASCII, so this should output 10, right?” Not so fast, my friend! Let’s break this down and see why JavaScript has other plans.
The Fundamentals at Play
1. Variables and Assignment
First things first, let’s talk about what’s happening with our variables:
var B = 65;
Β – We’re assigning a number to B. Simple enough!var grade = B;
Β – Here’s where people often get tripped up. We’re not assigning the letter ‘A’ to grade, we’re assigning the number 65.
Remember, JavaScript doesn’t automatically convert numbers to their ASCII character equivalents. It’s not that smart (or maybe it’s too smart, depending on how you look at it)!
2. The Switch Statement: JavaScript’s Picky Eater
Now, let’s talk about this switch statement. In JavaScript, switch statements use strict equality (===) for comparisons. This is crucial to understanding our puzzle.What’s strict equality, you ask? It’s JavaScript’s way of saying, “These two things must be exactly the same – same value AND same type.” It’s like trying to fit a square peg in a square hole; close doesn’t count.In our case:
grade
Β is 65 (a number)- Our cases are ‘A’, ‘B’, ‘C’ (strings)
See the mismatch? 65 === ‘A’ is false, and so are all the other comparisons. JavaScript doesn’t do any implicit type conversion here.
3. Type Coercion: The Missing Link
Speaking of type conversion, let’s chat about type coercion. It’s JavaScript’s way of converting values from one type to another. For example:
javascriptconsole.log(65 == '65'); // true
console.log(65 === '65'); // false
In the first line, JavaScript coerces the string ’65’ to a number. But in the second line (and in our switch statement), no coercion happens. This is why understanding the difference between == and === is super important in JavaScript!
4. ASCII: The Ghost in the Machine
ASCII (American Standard Code for Information Interchange) is a character encoding standard. In ASCII:
- ‘A’ is represented by the number 65
- ‘B’ is 66
- ‘C’ is 67
But here’s the kicker: JavaScript doesn’t automatically translate between ASCII values and their character representations. It’s like knowing someone’s phone number but not their name – you need to make the connection yourself.
The Solution: Making JavaScript Play Nice
Want to make our code work as expected? We need to explicitly convert our number to a string:
javascript var B = 65;
var grade = String.fromCharCode(B);
// Rest of the code stays the same
String.fromCharCode()
is our translator. It takes ASCII values and returns the corresponding characters. Now grade
will be ‘A’, and our switch statement will be happy!
The Bigger Picture: Why This Matters
Understanding these concepts isn’t just about acing interviews. It’s about writing better, more predictable JavaScript code. Here’s why:
- Type Safety: Knowing when JavaScript will and won’t convert types helps prevent bugs.
- Performance: Strict comparisons (===) are generally faster than loose ones (==).
- Readability: Code that’s explicit about types is easier for other developers (and future you) to understand.
Wrapping Up: The JavaScript Lesson
So, what have we learned on this wild ride through JavaScript land?
- Always be aware of your variable types.
- Switch statements are stricter than you might think.
- When in doubt, be explicit about type conversions.
- JavaScript’s quirks aren’t bugs; they’re features that keep us on our toes!
Remember, every “gotcha” moment in JavaScript is an opportunity to deepen your understanding. So next time you encounter a tricky bit of code, dive in, explore, and have fun unraveling the mystery!Keep coding, stay curious, and may your variables always be of the type you expect them to be! π»π