The ‘in’ Operator: JavaScript Interview Tips (Part 3)
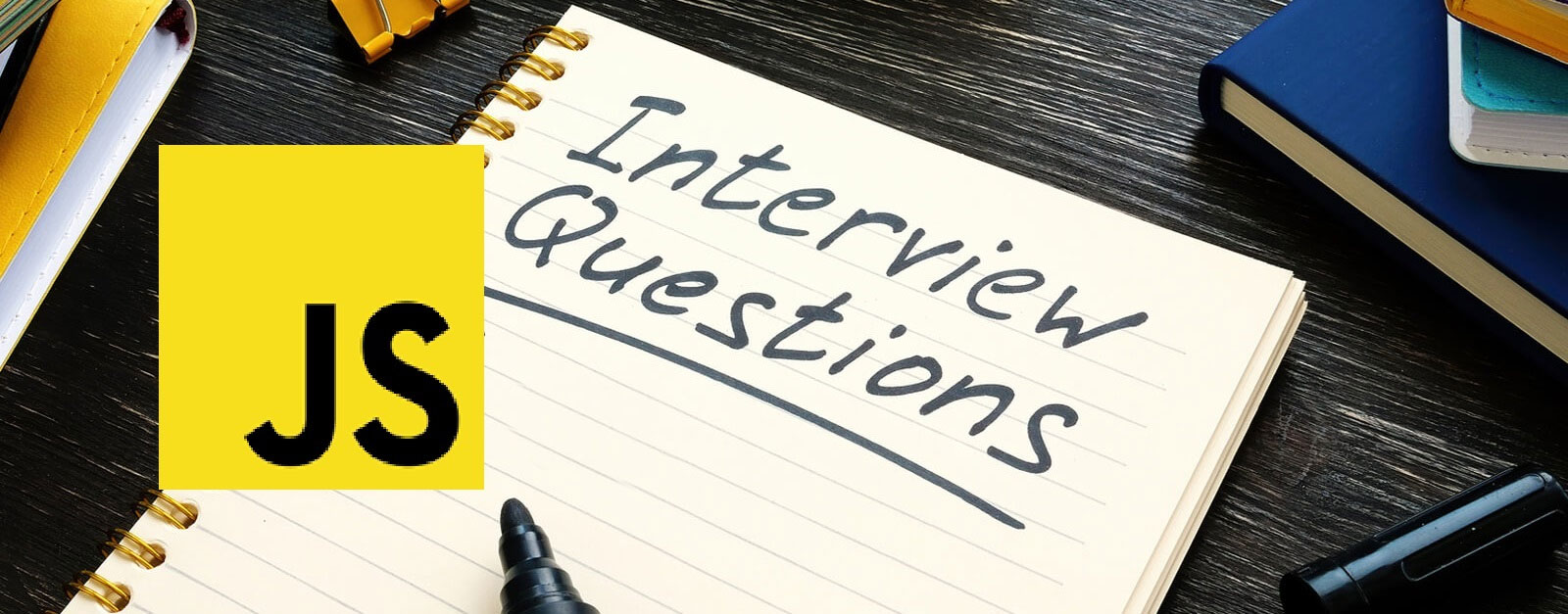
Hey there, fellow code enthusiasts! Ever found yourself scratching your head, wondering if a certain property exists in your JavaScript object? Well, you’re not alone. This is a common head-scratcher that even seasoned developers face, and it’s a hot topic in JavaScript interviews. So, let’s crack this mystery wide open!
Meet Your New Best Friend: The ‘in’ Operator
Imagine you’re at a party (a JavaScript object party, of course), and you’re trying to figure out if someone named “age” is there. The ‘in’ operator is like your reliable friend who knows everyone. You can simply ask, “Hey, is ‘age’ in this party?” and get a straightforward yes or no answer.Here’s how you’d do it in code:
const partyPeople = {
name: "JavaScript Jam",
location: "Code City",
attendees: 100
};
console.log('age' in partyPeople); // false (Aww, age didn't show up!)
console.log('location' in partyPeople); // true (Yep, location's here!)
How Does This Magic Work?
The ‘in’ operator is like a super-detective. It doesn’t just look at the guest list (the object’s own properties) but also checks if the person might be a plus-one (inherited properties). If it finds the name anywhere, it shouts “true!” Otherwise, it sadly reports “false.”
But Wait, There’s More!
Now, here’s a fun twist. The ‘in’ operator doesn’t care if someone actually showed up to the party. If their name is on the list, even if they’re marked as “undefined,” ‘in’ still says they’re there. Sneaky, right?
const mysteriousGuest = {
name: undefined
};
console.log('name' in mysteriousGuest); // true (They're on the list, even if they're a no-show!)
Other Ways to Check the Guest List
While ‘in’ is our star player, there are other methods to check who’s at the party:
- The Bouncer Method (hasOwnProperty()): This guy only checks the main guest list, ignoring any plus-ones.
- The “Are You There?” Method (=== undefined): This is like shouting a name and seeing if anyone responds. But be careful, sometimes people might be there but just not answer!
Why Should You Care?
Knowing how to use ‘in’ and its friends is like having a superpower in the JavaScript world. It helps you:
- Avoid embarrassing errors (like trying to talk to someone who’s not at the party)
- Make smart decisions based on who’s there
- Become the go-to person for solving object mysteries
Let’s examine each of the given options and see how they stack up:
1. exists
Verdict: IncorrectWhy it’s wrong: JavaScript doesn’t have a built-in ‘exists’ operator or method. If you’re coming from other programming languages, you might be tempted to think this exists (pun intended!), but it’s not a part of JavaScript’s syntax.Example of what doesn’t work:
const obj = { name: "JavaScript" };
console.log(exists obj.name); // This would cause a syntax error
2. in
Verdict: Correct!Why it’s right: The ‘in’ operator is JavaScript’s go-to tool for checking property existence. It works with both an object’s own properties and those inherited through its prototype chain.Let’s see it in action:
const language = { name: "JavaScript", year: 1995 };
console.log("name" in language); // true
console.log("version" in language); // false
// It even works with inherited properties
console.log("toString" in language); // true (inherited from Object.prototype)
3. within
Verdict: IncorrectWhy it’s wrong: ‘within’ is not a JavaScript operator or keyword. It might sound logical in English (“Is this property within this object?”), but JavaScript doesn’t recognize it.Example of what doesn’t work:
const framework = { name: "React" };
console.log("name" within framework); // This would cause a syntax error
4. exist
Verdict: IncorrectWhy it’s wrong: Similar to ‘exists’, ‘exist’ is not a valid JavaScript operator or method. It’s a common English word that makes sense in context, but it’s not part of JavaScript’s syntax.Example of what doesn’t work:
const feature = { async: true };
console.log(exist feature.async); // This would cause a syntax error
Why Understanding This is Crucial
Knowing the correct operator for property existence checking is vital for several reasons:
- Error Prevention: Using the correct operator helps you avoid runtime errors that could crash your application.
- Code Reliability: Proper property checking makes your code more robust, especially when dealing with dynamic data or APIs.
- Performance: The ‘in’ operator is optimized for property checking, potentially offering better performance than alternatives.
- Interview Success: This topic is a favorite in JavaScript interviews, so understanding it well can give you an edge.
Digging Deeper: The Power of ‘in’
The ‘in’ operator is more versatile than you might think:
- Array Indices: It works with array indices too!javascript
const arr = [1, 2, 3]; console.log(1 in arr); // true (index 1 exists) console.log(5 in arr); // false (index 5 doesn't exist)
- Inherited Properties: It checks the entire prototype chain.javascript
console.log("length" in []); // true (Array.prototype.length)
- Property vs. Value: Remember, ‘in’ checks for the property name, not its value.javascript
const obj = { prop: undefined }; console.log("prop" in obj); // true (the property exists, even though its value is undefined)
In Conclusion: The ‘in’ Operator Reigns Supreme
While ‘exists’, ‘within’, and ‘exist’ might seem logical in everyday language, in the world of JavaScript, ‘in’ is the undisputed champion for property existence checking. It’s not just about knowing the right keyword; it’s about understanding how JavaScript handles object properties and the nuances of property checking.So, the next time you need to check if a property exists in an object, remember: ‘in’ is in, and everything else is out! Happy coding, and may all your property checks be successful!