Mastering JavaScript Variables, Scope, and Hoisting
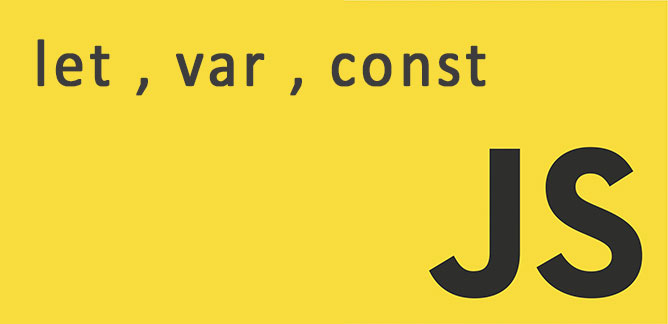
Introduction
JavaScript is a powerful and versatile programming language that forms the backbone of modern web development. Whether you’re a beginner or an experienced developer, understanding the nuances of JavaScript variables, scope, and hoisting is crucial for writing efficient and bug-free code. In this comprehensive guide, we’ll dive deep into these concepts, exploring their intricacies and providing practical examples to solidify your understanding.
Understanding JavaScript Variables
JavaScript variables are containers for storing data values. They are fundamental to any programming language, allowing developers to work with and manipulate data throughout their code. In JavaScript, there are three ways to declare variables: var
, let
, and const
.
The var
Keyword
The var
keyword was the original method for declaring variables in JavaScript. It has function scope or global scope, depending on where it’s declared. Here’s an example:
var greeting = "Hello, world!";
function sayHello() {
var message = "Welcome!";
console.log(message);
}
console.log(greeting); // Output: Hello, world!
console.log(message); // ReferenceError: message is not defined
In this example, greeting
is a global variable, while message
is function-scoped and only accessible within the sayHello
function.
The let
Keyword
Introduced in ES6, let
provides block-scoped variables. This means the variable is only accessible within the block it’s declared in, such as within an if
statement or a for
loop. For example:
let count = 0;
if (true) {
let count = 1;
console.log(count); // Output: 1
}
console.log(count); // Output: 0
The const
Keyword
Also introduced in ES6, const
is used to declare constants – variables whose values cannot be reassigned. Like let
, const
is block-scoped. Here’s an example:
const PI = 3.14159;
PI = 3; // TypeError: Assignment to a constant variable
It’s important to note that while const
prevents reassignment, it doesn’t make objects immutable. You can still modify the properties of a const
object.
Diving into JavaScript Scope
Scope in JavaScript refers to the current context of code, determining the accessibility of variables to JavaScript6. Understanding scope is crucial for writing maintainable and error-free code.
Global Scope
Variables declared outside any function or block have global scope. They can be accessed from anywhere in the JavaScript program. For example:
var globalVar = "I'm global!";
function testScope() {
console.log(globalVar); // Output: I'm global!
}
testScope();
console.log(globalVar); // Output: I'm global!
Local Scope
Variables declared within a function have local scope. They are only accessible within that function. For example:
function testLocalScope() {
var localVar = "I'm local!";
console.log(localVar); // Output: I'm local!
}
testLocalScope();
console.log(localVar); // ReferenceError: localVar is not defined
Block Scope
Introduced with let
and const
in ES6, block scope limits the variable’s scope to the block, statement, or expression it was declared in. For example:
if (true) {
let blockVar = "I'm block-scoped!";
console.log(blockVar); // Output: I'm block-scoped!
}
console.log(blockVar); // ReferenceError: blockVar is not defined
Unraveling JavaScript Hoisting
Hoisting is a JavaScript mechanism where variables and function declarations are moved to the top of their respective scopes during the compile phase, before the code is executed. This can lead to some unexpected behavior if not properly understood.
Variable Hoisting
When using var
, variable declarations are hoisted to the top of their scope, but not their initializations. For example:
console.log(x); // Output: undefined
var x = 5;
This is equivalent to:
var x;
console.log(x); // Output: undefined
x = 5;
It’s important to note that let
and const
declarations are also hoisted, but they are not initialized. Accessing them before the declaration results in a ReferenceError.
Function Hoisting
Function declarations are also hoisted in JavaScript. This means you can call a function before it’s declared in your code:
sayHello(); // Output: Hello, world!
function sayHello() {
console.log("Hello, world!");
}
However, function expressions are not hoisted:
sayHello(); // TypeError: sayHello is not a function
var sayHello = function() {
console.log("Hello, world!");
};
Best Practices for Working with Variables, Scope, and Hoisting
Understanding these concepts is crucial, but knowing how to apply them effectively is equally important. Here are some best practices to keep in mind:
- Use
const
by default: If you don’t need to reassign a variable, useconst
. This makes your intentions clear and helps prevent accidental reassignments. - Use
let
for variables that will be reassigned: When you need to reassign a variable, uselet
. This provides block scoping and helps prevent unintended side effects. - Avoid using
var
: In modern JavaScript development, it’s generally better to uselet
andconst
instead ofvar
. This provides more predictable scoping behavior. - Declare variables at the top of their scope: This practice, known as hoisting, can help prevent confusion and make your code more readable.
- Be aware of the temporal dead zone: When using
let
andconst
, be aware that accessing these variables before their declaration will result in a ReferenceError. - Use immediately invoked function expressions (IIFE) for encapsulation: IIFEs can help you create private scopes and avoid polluting the global namespace.
Conclusion
JavaScript variables, scope, and hoisting are fundamental concepts that every JavaScript developer should master. By understanding how these mechanisms work, you can write more efficient, maintainable, and bug-free code. Remember, practice is key to internalizing these concepts. Experiment with different scenarios, and don’t be afraid to make mistakes – they’re often the best teachers. As you continue your JavaScript journey, keep exploring and learning. The language is constantly evolving, and staying up-to-date with the latest features and best practices will help you become a more proficient developer.