Master JavaScript Equality and Null Checks: Interview Tips (Part 1)
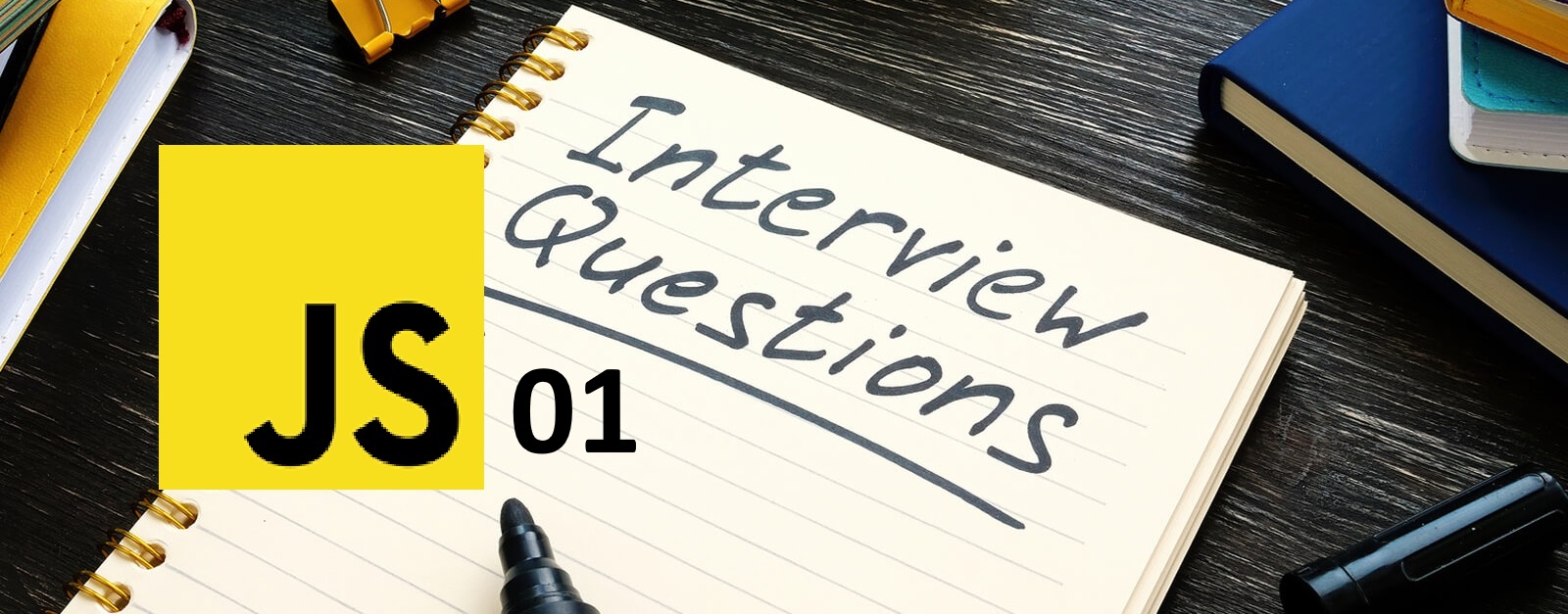
Cracking the Code: JavaScript’s Tricky Null Checks Explained (Part 1)
Hey there, fellow code enthusiasts! 👋 Welcome to the first installment of our “JavaScript Interview Questions” series. Today, we’re diving into a topic that’s as common as coffee in a developer’s life: checking for null values in JavaScript. Trust me, it’s more exciting than it sounds!
The Question That Stumps Even the Pros
Picture this: You’re in an interview, palms sweaty, code editor ready. The interviewer hits you with this seemingly simple question:
Which of these bad boys correctly checks if “a” is not equal to “null”?
if(a!null)
if (!a)
if(a!==null)
if(a!=null)
Looks easy, right? Well, hold onto your keyboards, because we’re about to unpack why this question is trickier than trying to explain what you do as a developer to your grandparents.
Breaking It Down: The Good, The Bad, and The Ugly
1. if(a!null)
– The Syntax Rebel
Oh boy, this one’s like showing up to a formal dinner in your pajamas. It’s just… wrong. JavaScript’s like, “Dude, where’s my operator?” Always remember, in JS, we need to play by the rules (at least some of them).
2. if (!a)
– The Overeager Bouncer
This guy’s a bit too enthusiastic. It’s not just checking for null; it’s kicking out everything that’s falsy. Null, undefined, empty strings, zero – they’re all getting the boot. It’s like using a sledgehammer to crack a nut.
3. if(a!==null)
– The Strict Security Guard
Now we’re talking! This is the bouncer who checks IDs thoroughly. It’s not just looking at the value; it’s making sure the type matches too. It’s precise, it’s strict, it’s what you want in most cases.
4. if(a!=null)
– The Laid-back Lookout
This one’s correct, but it’s got a bit of a “meh, close enough” attitude. It’ll let undefined
slip through like it’s null’s cooler cousin. Sometimes that’s okay, but it can lead to some head-scratching moments later.
The Winner and Why It’s Your New Best Friend
Drumroll, please! 🥁 The champion is:
if(a !== null)
Why? Because it’s like that friend who always tells you if you’ve got spinach in your teeth. It’s honest, direct, and doesn’t mess around with type coercion shenanigans.
Equality in JavaScript: A Tale of Two Operators
JavaScript’s equality operators are like twins with very different personalities:
Loose Equality (==
and !=
) – The Easygoing Twin
This one’s all about “vibes.” It’ll try to make things work, converting types left and right. It’s like that friend who says, “Close enough!” a lot. Sometimes helpful, often confusing.
null == undefined // "Yeah, they're basically the same, right?"
1 == '1' // "One, won, same difference!"
Strict Equality (===
and !==
) – The Perfectionist Twin
This twin’s all about precision. No type conversion, no assumptions. It’s like your friend who insists on measuring ingredients to the gram when baking.
null === undefined // "Nope, they're different!"
1 === '1' // "A number and a string? No way, José!"
null and undefined: The Odd Couple of JavaScript
Think of null
as deliberately empty, like an empty box you’re saving for later. undefined
is more like, “Oops, I forgot to put something here.”
They’re different, but sometimes JavaScript treats them like they’re the same, especially with loose equality. It’s like twins who dress differently but still finish each other’s sentences.
Best Practices: Your Code’s Best Friends
- Stick to Strict: Use
!==
for null checks. It’s like using a spell-checker – catches more mistakes. - Be Crystal Clear: If you need to check for both null and undefined, just say so:
if (a !== null && a !== undefined) {
// Now we're cooking with gas!
}
- The Nullish Coalescing Operator (
??
) is Your Pal: It’s great for default values:
const username = inputUsername ?? 'Guest';
// If inputUsername is null or undefined, we'll go with 'Guest'
- Optional Chaining (
?.
) to the Rescue: It’s like a safety net for your object properties:
const middleName = user?.name?.middle;
// No more "Cannot read property 'middle' of undefined" nightmares!
Real-world Scenarios: Where This Stuff Actually Matters
- Dealing with Flaky APIs: When that API response is as unpredictable as weather forecasts.
- Form Validation: Making sure users actually type something in that “required” field.
- Database Queries: When your database returns null, and you need to handle it gracefully.
- Config Files: Because sometimes, no config is better than a broken config.
Wrapping Up
And there you have it, folks! We’ve journeyed through the land of null checks and equality in JavaScript. Remember, in the world of coding, being null ain’t nothing to mess with. Always check your nulls, be strict with your equality, and your code will thank you (probably not literally, but you get the idea).
Stay tuned for our next episode, where we’ll tackle more JavaScript quirks that make interviewers grin and developers sweat. Until then, keep your code clean and your console errors to a minimum! Happy coding! 🚀👨💻👩💻