Master React State Management: Simple Tips with useState, Spread Operator, & prevState
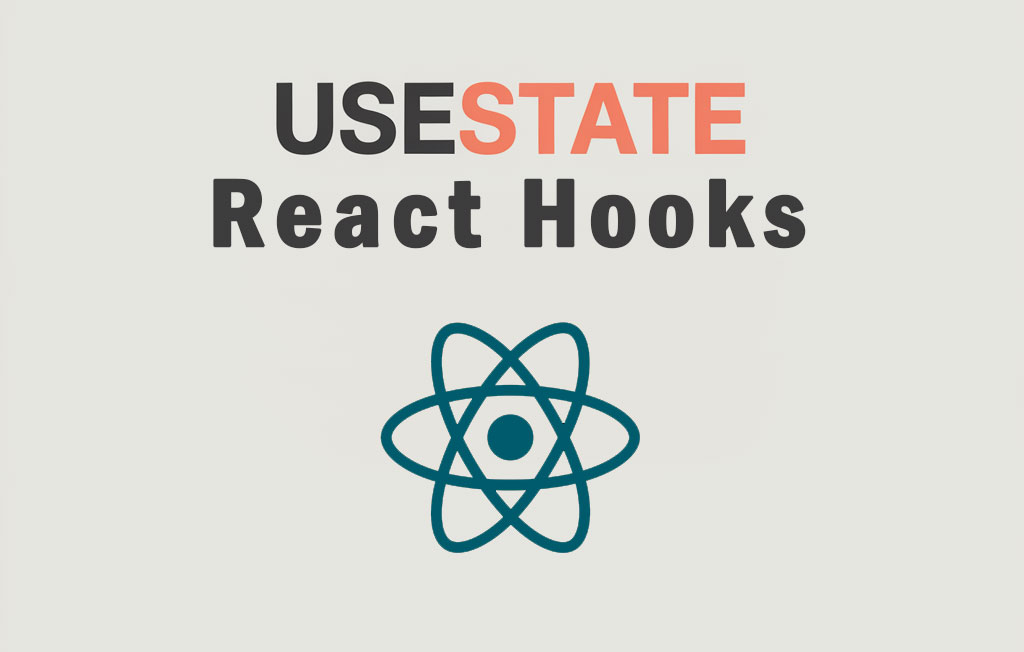
In the ever-evolving world of web development, React has emerged as a powerhouse for building dynamic and interactive user interfaces. At the heart of React’s efficiency lies its state management system, which allows developers to create responsive and data-driven applications. In this comprehensive guide, we’ll dive deep into three essential concepts that every React developer should master: useState, the spread operator (…), and prevState. We’ll also explore the crucial role of setState in React applications.
Understanding useState in React
What is useState?
useState is a React Hook that allows functional components to manage state. It provides a way to add state to your components without converting them to class components.
Syntax and Usage
const [state, setState] = useState(initialState);
Here’s a breakdown of the syntax:
state
: The current state valuesetState
: A function to update the stateinitialState
: The initial value of the state
Example: Counter Component
Let’s create a simple counter component to demonstrate useState:
import React, { useState } from 'react';
function Counter() {
const [count, setCount] = useState(0);
return (
<div>
<p>You clicked {count} times</p>
<button onClick={() => setCount(count + 1)}>
Click me
</button>
</div>
);
}
In this example, we initialize the count state to 0 and update it using the setCount function when the button is clicked.
Benefits of useState
- Simplicity: useState provides a straightforward way to add state to functional components.
- Readability: It makes the code more readable and easier to understand.
- Performance: React optimizes useState for performance, making it efficient for most use cases.
The Spread Operator (…) in React
What is the Spread Operator?
The spread operator (…) is a powerful feature in JavaScript that allows an iterable (like an array or object) to be expanded into individual elements.
Using Spread Operator with Arrays
const arr1 = [1, 2, 3];
const arr2 = [...arr1, 4, 5]; // [1, 2, 3, 4, 5]
Using Spread Operator with Objects
const obj1 = { a: 1, b: 2 };
const obj2 = { ...obj1, c: 3 }; // { a: 1, b: 2, c: 3 }
Spread Operator in React State Updates
The spread operator is particularly useful when updating state in React, especially for objects and arrays.
const [user, setUser] = useState({ name: 'John', age: 30 });
// Updating a property
setUser({ ...user, age: 31 });
This creates a new object with all properties of the original user object, but with the age property updated.
Understanding prevState in React
What is prevState?
prevState refers to the previous state value in React. It’s particularly useful when you need to update state based on its previous value.
Why Use prevState?
Using prevState ensures that you’re working with the most up-to-date state value, especially in scenarios where state updates may be batched or asynchronous.
Example: Counter with prevState
function Counter() {
const [count, setCount] = useState(0);
const increment = () => {
setCount(prevCount => prevCount + 1);
};
return (
<div>
<p>Count: {count}</p>
<button onClick={increment}>Increment</button>
</div>
);
}
In this example, we use prevCount to ensure that we’re always incrementing based on the latest count value.
Combining useState, Spread Operator, and prevState
Let’s look at a more complex example that combines all these concepts:
function UserProfile() {
const [user, setUser] = useState({
name: 'John Doe',
email: 'john@example.com',
preferences: {
theme: 'light',
notifications: true
}
});
const updateTheme = () => {
setUser(prevUser => ({
...prevUser,
preferences: {
...prevUser.preferences,
theme: prevUser.preferences.theme === 'light' ? 'dark' : 'light'
}
}));
};
return (
<div>
<h2>{user.name}</h2>
<p>Email: {user.email}</p>
<p>Theme: {user.preferences.theme}</p>
<button onClick={updateTheme}>Toggle Theme</button>
</div>
);
}
In this example:
- We use useState to manage a complex user object.
- The spread operator is used to create a new object while updating nested properties.
- prevUser ensures we’re working with the most current state when updating.
Why Use setState in React?
The Role of setState
setState is a method used in class components to update the component’s state. In functional components, it’s replaced by the setter function returned by useState.
Key Reasons to Use setState:
- Triggering Re-renders: When setState is called, React knows that the component’s state has changed and that it needs to re-render the component.
- Batching Updates: React may batch multiple setState calls for performance reasons. Using setState ensures that all updates are properly queued and applied.
- Asynchronous Nature: setState operations are asynchronous. React may delay the update for performance optimization.
- Partial Updates: setState allows you to update only specific parts of the state without affecting others.
Example: setState in Class Component
class Counter extends React.Component {
constructor(props) {
super(props);
this.state = { count: 0 };
}
increment = () => {
this.setState(prevState => ({ count: prevState.count + 1 }));
}
render() {
return (
<div>
<p>Count: {this.state.count}</p>
<button onClick={this.increment}>Increment</button>
</div>
);
}
}
setState vs useState
While setState is used in class components, useState serves a similar purpose in functional components. The key differences are:
- Syntax: useState is more concise and doesn’t require binding.
- Multiple States: useState allows for multiple state variables in a single component.
- No This Keyword: Functional components with useState don’t rely on the ‘this’ keyword.
Best Practices and Tips
- Use Functional Updates: When the new state depends on the previous state, always use the functional form of setState or useState.javascript
setCount(prevCount => prevCount + 1);
- Avoid Direct State Mutations: Always create a new object or array when updating state, especially with complex data structures.javascript
// Incorrect const newUser = user; newUser.name = 'Jane'; setUser(newUser); // Correct setUser({ ...user, name: 'Jane' });
- Keep State Updates Simple: Break down complex state updates into smaller, more manageable pieces.
- Use Multiple State Variables: Instead of a single large state object, consider using multiple useState calls for unrelated pieces of state.
- Optimize Re-renders: Use React.memo or useMemo for expensive computations to prevent unnecessary re-renders.
Common Pitfalls and How to Avoid Them
- Updating State Directly: Never modify state directly. Always use setState or the setter function from useState.
- Assuming Immediate Updates: Remember that state updates may be asynchronous. Don’t rely on the state being updated immediately after calling setState.
- Forgetting to Use Spread Operator: When updating objects or arrays in state, always create a new copy using the spread operator to ensure immutability.
- Overusing State: Not everything needs to be in state. Use local variables for values that don’t affect the render output.
- Ignoring prevState: When updating state based on its previous value, always use the functional update form to ensure you’re working with the most current state.
Advanced Scenarios
Managing Complex State
For more complex state management scenarios, consider using:
- useReducer: A more powerful alternative to useState for complex state logic.
- Context API: For sharing state across multiple components without prop drilling.
- State Management Libraries: Like Redux or MobX for large-scale applications with complex state interactions.
Custom Hooks
Create custom hooks to encapsulate and reuse stateful logic across components:
function useCounter(initialCount = 0) {
const [count, setCount] = useState(initialCount);
const increment = () => setCount(prevCount => prevCount + 1);
const decrement = () => setCount(prevCount => prevCount - 1);
return { count, increment, decrement };
}
// Usage
function Counter() {
const { count, increment, decrement } = useCounter();
return (
<div>
<p>Count: {count}</p>
<button onClick={increment}>+</button>
<button onClick={decrement}>-</button>
</div>
);
}
Conclusion
Mastering useState, the spread operator, and prevState is crucial for effective React development. These tools, combined with a solid understanding of why we use setState, provide a powerful framework for managing state in React applications. By following best practices and avoiding common pitfalls, you can create more efficient, maintainable, and scalable React components.Remember, state management in React is an art as much as it is a science. It requires practice, experimentation, and a deep understanding of React’s principles. As you continue to work with these concepts, you’ll develop an intuition for when and how to use them most effectively.